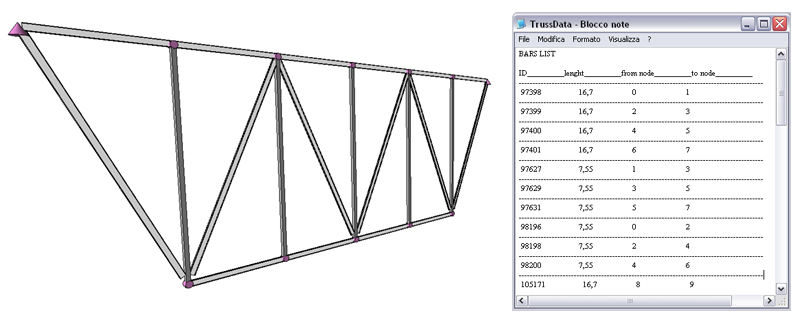
Thanks to prof. Todesco for his vital help.
FileInfo t = new FileInfo(@"C:\Documents and Settings\Gguarnieri\Documenti\Desktop\TrussData.txt");
StreamWriter Tex = t.CreateText();
Tex.WriteLine("BARS LIST");
Tex.Write(Tex.NewLine);
Tex.Write("{0}_________", "ID");
Tex.Write("{0}_________", "lenght");
Tex.Write("{0}_________", "from node");
Tex.Write("{0}_________", "to node");
Tex.Write(Tex.NewLine);
Tex.Write("-----------------------------------------------------------------------------------------");
Tex.Write(Tex.NewLine);
SetVertici vertici = new SetVertici();
double ConvMeter = 0.3048;
int approx = 2;
int numBars = 0;
Level level=null;
ElementIterator levelIter = Application.ActiveDocument.get_Elements(typeof(Level));
levelIter.Reset();
while (levelIter.MoveNext())
{
Level level1 = levelIter.Current as Level;
if (level1.Elevation > 0)
{
level = level1;
}
}
ElementIterator elemIter = Application.ActiveDocument.get_Elements(typeof(FamilyInstance));
elemIter.Reset();
while (elemIter.MoveNext())
{
FamilyInstance FamInst = elemIter.Current as FamilyInstance;
StructuralType StructType = FamInst.StructuralType;
Location Loc = FamInst.Location;
ElementId ElemId = FamInst.Id;
string familyName = FamInst.Name;
string NameFam = ElemId.Value.ToString();
int giunto = 0;
Vertice v = null;
if (familyName == "cone")
{
giunto = 1;
LocationPoint point = Loc as LocationPoint;
v = vertici.getVertice(point.Point,giunto);
}
else if (familyName == "cylinder")
{
giunto = 2;
LocationPoint point = Loc as LocationPoint;
v = vertici.getVertice(point.Point,giunto);
}
else if (StructType == StructuralType.Beam || StructType == StructuralType.Brace)
{
numBars = ++numBars;
LocationCurve CurveLoc = Loc as LocationCurve;
Curve curve = CurveLoc.Curve;
XYZ StartPoint = curve.get_EndPoint(0);
XYZ EndPoint = curve.get_EndPoint(1);
Vertice v0 = vertici.getVertice(StartPoint, giunto);
string id0 = v0.id.ToString();
Vertice v1 = vertici.getVertice(EndPoint, giunto);
string id1 = v1.id.ToString();
string lenght = (Math.Round((curve.Length) * ConvMeter, approx)).ToString();
string data = String.Format(" {0} {1,16} {2,15} {3,20}", NameFam, lenght, id0,id1);
Tex.WriteLine(data);
Tex.WriteLine("-----------------------------------------------------------------------------------------");
}
else if(StructType== StructuralType.Column)
{
numBars = ++numBars;
LocationPoint point = Loc as LocationPoint;
Vertice v0 = vertici.getVertice(point.Point, giunto);
string id0 = v0.id.ToString();
Vertice v1 = vertici.getVertice(v0.x,v0.y,level.Elevation,giunto);
string id1 = v1.id.ToString();
string lenght = (Math.Round((v0.distance(v1)) * ConvMeter, approx)).ToString();
string data = String.Format(" {0} {1,16} {2,15} {3,20}", NameFam, lenght, id0, id1);
Tex.WriteLine(data);
Tex.WriteLine("-----------------------------------------------------------------------------------------");
}
}
Tex.Write(Tex.NewLine);
Tex.WriteLine("NODES LIST");
Tex.Write(Tex.NewLine);
Tex.Write("{0}_________", "NUMBER");
Tex.Write("{0,5}_________", "X");
Tex.Write("{0,5}_________", "Y");
Tex.Write("{0,5}_________", "Z");
Tex.Write("{0,5}_________", "JOINT");
Tex.Write(Tex.NewLine);
Tex.Write("-----------------------------------------------------------------------------------------");
Tex.Write(Tex.NewLine);
for (int i = 0; i < vertici.Count(); i++)
{
Vertice v = vertici.at(i);
string giunto = "";
if (v.joint == 0)
{
giunto = "unknown";
}
else if (v.joint == 1)
{
giunto = "cone";
}
else if (v.joint == 2)
{
giunto = "cylinder";
}
Tex.Write("{0,3}", v.id.ToString());
Tex.Write("{0,20}", (Math.Round((v.x) * ConvMet, approx)).ToString());
Tex.Write("{0,20}", (Math.Round((v.y) * ConvMet, approx)).ToString());
Tex.Write("{0,20}", (Math.Round((v.z) * ConvMet, approx)).ToString());
Tex.Write("{0,20}", giunto);
Tex.Write(Tex.NewLine);
Tex.Write("-----------------------------------------------------------------------------------------");
Tex.Write(Tex.NewLine);
}
Tex.Write(Tex.NewLine);
Tex.Write("{0,10}_________", "BARS number");
Tex.Write("{0,10}_________", numBars.ToString());
Tex.Write("{0,10}_________", "NODES number");
Tex.Write("{0,10}_________", vertici.Count().ToString());
Tex.Close();
}
public class SetVertici
{
List<Vertice> vertici = new List<Vertice>();
public Vertice getVertice(double x, double y, double z, int joint)
{
Vertice posizione = new Vertice(x, y, z, joint);
Vertice piuVicino = null;
double distanza2Minima = 0;
for (int i = 0; i < vertici.Count(); i++)
{
double distanza2 = vertici[i].distance2(posizione);
int giuntoVero = vertici[i].joint;
if (giuntoVero != 0 && distanza2<5)
{
posizione.joint = giuntoVero;
}
if (piuVicino == null || distanza2 < distanza2Minima)
{
piuVicino = vertici[i];
distanza2Minima = distanza2;
}
}
if (piuVicino != null && distanza2Minima < 1)
{
piuVicino.joint = posizione.joint;
return piuVicino;
}
posizione.id = vertici.Count();
vertici.Add(posizione);
return posizione;
}
public Vertice getVertice(Autodesk.Revit.Geometry.XYZ coords,int tipoGiunto)
{
return getVertice(coords.X, coords.Y, coords.Z,tipoGiunto);
}
public int Count()
{
return vertici.Count();
}
public Vertice at(int index)
{
return vertici[index];
}
}
public class Vertice
{
double _x;
double _y;
double _z;
int _id;
int _joint;
public Vertice(double x, double y, double z, int TipoGiunto)
{
_x = x;
_y = y;
_z = z;
_id = -1;
_joint = TipoGiunto;
}
public double x
{
get { return _x; }
}
public double y
{
get { return _y; }
}
public double z
{
get { return _z; }
}
public double distance2(Vertice v)
{
return Math.Pow(v.x - _x, 2) + Math.Pow(v.y - _y, 2) + Math.Pow(v.z - _z, 2);
}
public double distance(Vertice v)
{
return Math.Sqrt(distance2(v));
}
public int id
{
get { return _id; }
set { _id = value; }
}
public int joint
{
get { return _joint; }
set { _joint = value; }
}
}